PhpStorm: Short hand syntax for arrays
Since version 5.4 PHP is capable of using a short hand syntax for arrays. But how to enforce this in PhpStorm?
In some cases it is necessary that you have to pass function parameters as an array. Especially when you work with nested arrays the php array function tends to make the code looking ugly. If you take a look at other languages (Ruby, Python, Javascript) there is a short syntax for arrays using square brackets.
In PHP 5.4.0 this behaviour was adopted to make code more readable and maintainable.
The following statement couples are the same
$fruits = array(0 => 'apple', 1 => 'orange');
$fruits = [0 => 'apple', 1 => 'orange'];
$fruits = array('apple', 'orange');
$fruits = ['apple', 'orange'];
insertFruits(array(0 => 'apple', 1 => 'orange'));
insertFruits([0 => 'apple', 1 => 'orange']);
The TYPO3 core is currently using both syntaxes, but the short hand syntax is the preferred one for new features or when rewriting existing code.
I'm a developer since ages and started programming in PHP in 1998 and I'm used to using the longer syntax. So if I don't set my IDE to a setting where the short hand syntax is set to preferred, I'll have a mixture of both.
Since the traditional syntax is still allowed, PhpStorm can't be configured to autocorrect the old traditional one to the new short hand syntax. However, it can show you a warning using inspections when using the traditional syntax. PhpStorm can also be configured to replace the array() constructs with [] in array declarations during reformatting.
Configure
Go to the 'Preferences' and open 'Editors'. In here there are two parts where you can configure the behaviour of PhpStorm regarding short hand syntax for arrays.
First open 'Code Style > PHP'. On the tab 'Other' check the option 'Force short declaration style' under 'Array declaration style'. This option will be used for reformatting.
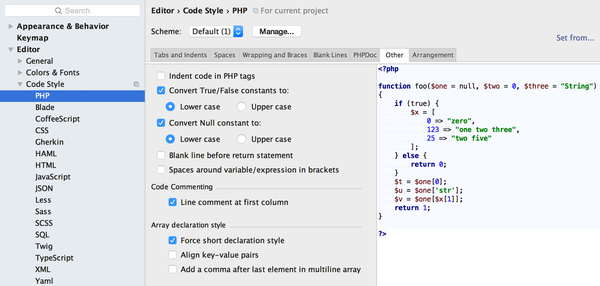
Secondly open 'Inspections', scroll down to 'PHP', open it and open the option 'Code Style'. Then check the box behind 'Traditional syntax array literal detected'. This option will be used by the inspector.
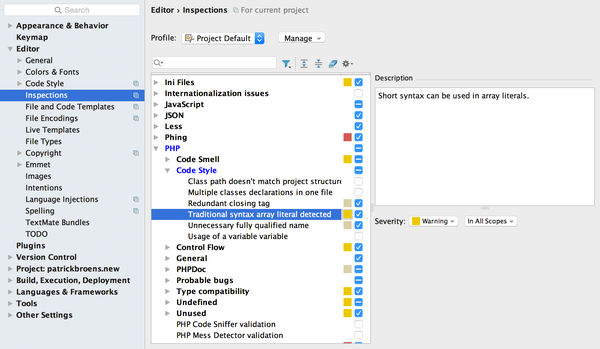
Inspector
It depends on how your inspector is configured in PhpStorm, but I let the inpector check my work on the fly, which means while I'm typing.
While typing your code and inserting the traditional syntax PhpStorm will show you a warning. See the yellow/brownish color as background color of the word 'array?
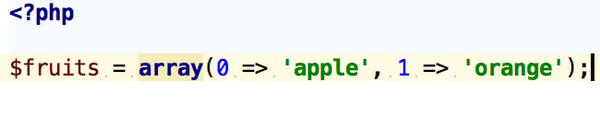
Hovering this warning it shows you a message box, stating what the warning exactly is about. In this case 'Traditional syntax array literal detected'.
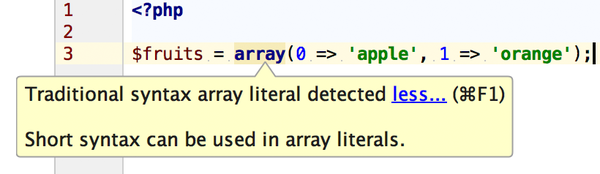
There is also the possibility to inspect the code of your whole project, the current file or a custom scope. To do so click 'Code > Inspect Code' in the main menu of PhpStorm. A popup box will appear where you can select a certain scope. Be aware that big projects might take some time to inspect, depending on the amount of inspectors checked in the preferences of PhpStorm.
The inspector will show a list of all warnings, errors and other problems it could find. In case of my example, there is only one: Traditional syntax array literal detected.
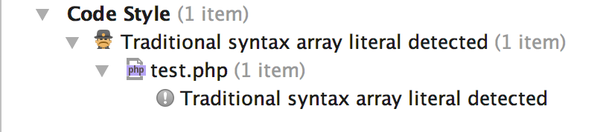
Right clicking on the warning - you can select more warnings if you like - will show you an option menu. Select 'Convert Array Syntax To Short' to correct the code.
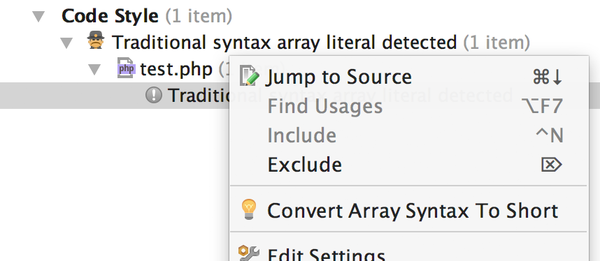
Reformat
PhpStorm lets you reformat source code to meet the requirements of your code style. PhpStorm will lay out spacing, indents, keywords etc. Reformatting can apply to the selected text, entire file, or entire project.
There are several options to reformat your code. Again this can be a file, a directory (with or without subdirectories) or just a selected part of your code. One option is to have the cursor somewhere in your code and select 'Code > Reformat Code'. This will reformat the current file. If your settings are right, your traditional arry syntax has now changed to the short hand syntax